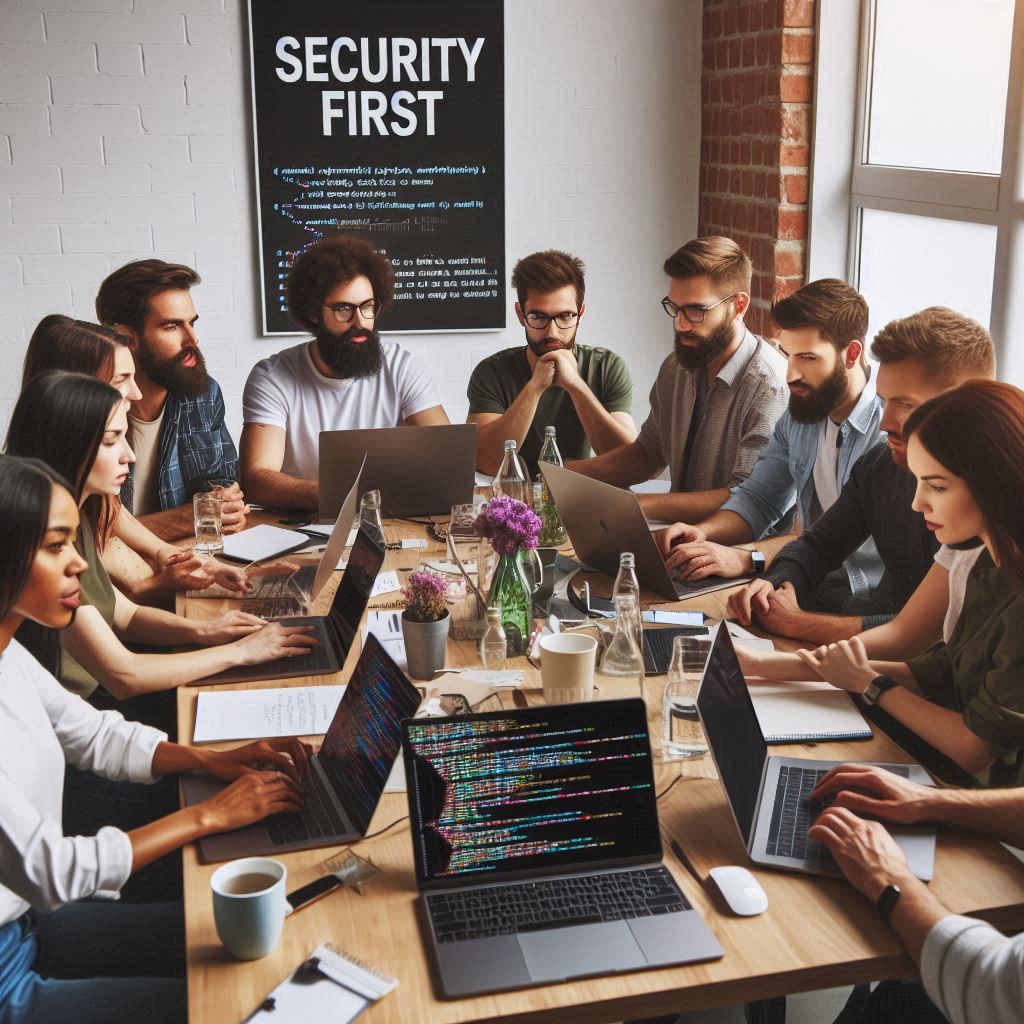
Securing Django Applications: Best Practices and Common Risks
August 20, 2024Django, a powerful web framework for Python, is designed with security in mind. However, as with any web application, Django projects can be vulnerable to various security threats if not properly configured and maintained. This article will explore best practices for securing Django applications, discuss common security risks, and provide guidance on protecting your application from vulnerabilities.
Understanding Django's Built-in Security Features
Before delving into specific security measures, it's essential to understand that Django comes with several built-in security features:
- Cross-site scripting (XSS) protection
- Cross-site request forgery (CSRF) protection
- SQL injection prevention
- Clickjacking protection
- Host header validation
- SSL/HTTPS support
While these features provide a solid foundation for security, developers must still take additional steps to ensure their Django applications are fully protected.
Best Practices for Securing Django Applications
- Keep Django and Dependencies Updated
One of the most critical security practices is keeping Django and all its dependencies up to date. Regularly check for and apply security updates to mitigate known vulnerabilities. Use tools like 'pip-audit' to scan for known vulnerabilities in your project's dependencies.
- Properly Configure Settings
Ensure that your Django settings are correctly configured for a production environment:
- Set DEBUG = False in production
- Use strong, unique SECRET_KEY values
- Configure ALLOWED_HOSTS to restrict which hosts can serve your application
- Enable SECURE_SSL_REDIRECT to force HTTPS
- Set SESSION_COOKIE_SECURE and CSRF_COOKIE_SECURE to True
- Implement Strong Authentication
Use Django's built-in authentication system and consider implementing two-factor authentication (2FA) for added security. Enforce strong password policies and implement account lockout mechanisms to prevent brute-force attacks.
- Manage User Permissions Carefully
Utilise Django's built-in user and group permissions system to control access to different parts of your application. Implement the principle of least privilege, granting users only the permissions they need to perform their tasks.
- Secure File Uploads
If your application allows file uploads, implement strict validation and sanitisation of uploaded files. Store uploaded files outside the web root and use Django's FileField with proper permissions.
- Implement Content Security Policy (CSP)
Use Django-CSP or similar middleware to implement a Content Security Policy, which helps prevent XSS attacks by specifying which sources of content are allowed to be loaded.
- Use Secure Cookies
Ensure that all cookies are marked as 'secure' and 'httponly' to prevent them from being accessed by client-side scripts or transmitted over unsecured connections.
- Implement Rate Limiting
Use Django-ratelimit or similar tools to implement rate limiting on views, especially those handling user authentication or form submissions, to prevent brute-force attacks and DoS attempts.
- Secure Database Connections
Use SSL/TLS for database connections and store database credentials securely, preferably using environment variables rather than hardcoding them in settings files.
- Implement Proper Logging and Monitoring
Set up comprehensive logging for your Django application, including security-related events. Regularly review logs and implement monitoring solutions to detect and respond to potential security incidents promptly.
Common Security Risks in Django Applications
While Django provides robust security features, several common risks can still affect Django applications:
- Insufficient Input Validation
Failing to properly validate and sanitise user input can lead to various security vulnerabilities, including XSS and SQL injection attacks.
- Misconfigured Settings
Improperly configured Django settings, such as leaving DEBUG mode enabled in production or using weak SECRET_KEY values, can expose sensitive information and weaken application security.
- Insecure Direct Object References (IDOR)
IDOR vulnerabilities occur when an application exposes references to internal implementation objects, allowing attackers to manipulate these references to access unauthorised data.
- Broken Authentication and Session Management
Weak authentication mechanisms, improper session handling, or failure to implement secure password storage can lead to compromised user accounts.
- Security Misconfiguration
Misconfigured web servers, databases, or Django itself can lead to security vulnerabilities. This includes issues like leaving default accounts enabled or exposing sensitive files.
- Sensitive Data Exposure
Failing to properly encrypt sensitive data or transmitting it over insecure channels can lead to data breaches.
- Cross-Site Request Forgery (CSRF)
While Django provides CSRF protection, improper implementation or disabling this feature can leave applications vulnerable to CSRF attacks.
- Using Components with Known Vulnerabilities
Failing to keep Django and its dependencies updated can expose applications to known security vulnerabilities.
Protecting Your Django Application from Vulnerabilities
To protect your Django application from these and other vulnerabilities, consider the following measures:
- Implement Robust Input Validation
Use Django's form validation features and implement additional server-side validation to ensure all user input is properly sanitised and validated before processing.
- Use Django's ORM and Parameterised Queries
Leverage Django's Object-Relational Mapping (ORM) system and use parameterised queries to prevent SQL injection attacks.
- Implement Proper Authentication and Authorization
Use Django's built-in authentication system and implement proper authorization checks throughout your application. Consider using decorators like @login_required and @permission_required to enforce access controls.
- Encrypt Sensitive Data
Use strong encryption for sensitive data both at rest and in transit. Implement HTTPS across your entire site and use Django's built-in cryptographic signing features when necessary.
- Implement Security Headers
Use security headers like X-Frame-Options, X-XSS-Protection, and Strict-Transport-Security to enhance your application's security posture.
- Conduct Regular Security Audits
Perform regular security audits of your Django application, including code reviews, vulnerability scans, and penetration testing.
- Use Django Security Packages
Leverage additional Django security packages like django-secure, django-axes, and django-honeypot to enhance your application's security features.
- Implement Proper Error Handling
Ensure that your application handles errors gracefully and doesn't expose sensitive information in error messages or stack traces.
Conclusion
Securing a Django application is an ongoing process that requires vigilance and a proactive approach. By following the best practices outlined in this article, understanding common security risks, and implementing appropriate protective measures, you can significantly enhance the security of your Django application.
Remember that security is not a one-time task but a continuous process. Stay informed about the latest security threats and Django security updates, and regularly review and update your application's security measures. By prioritising security throughout the development lifecycle, you can build robust, secure Django applications that protect your users' data and maintain their trust.