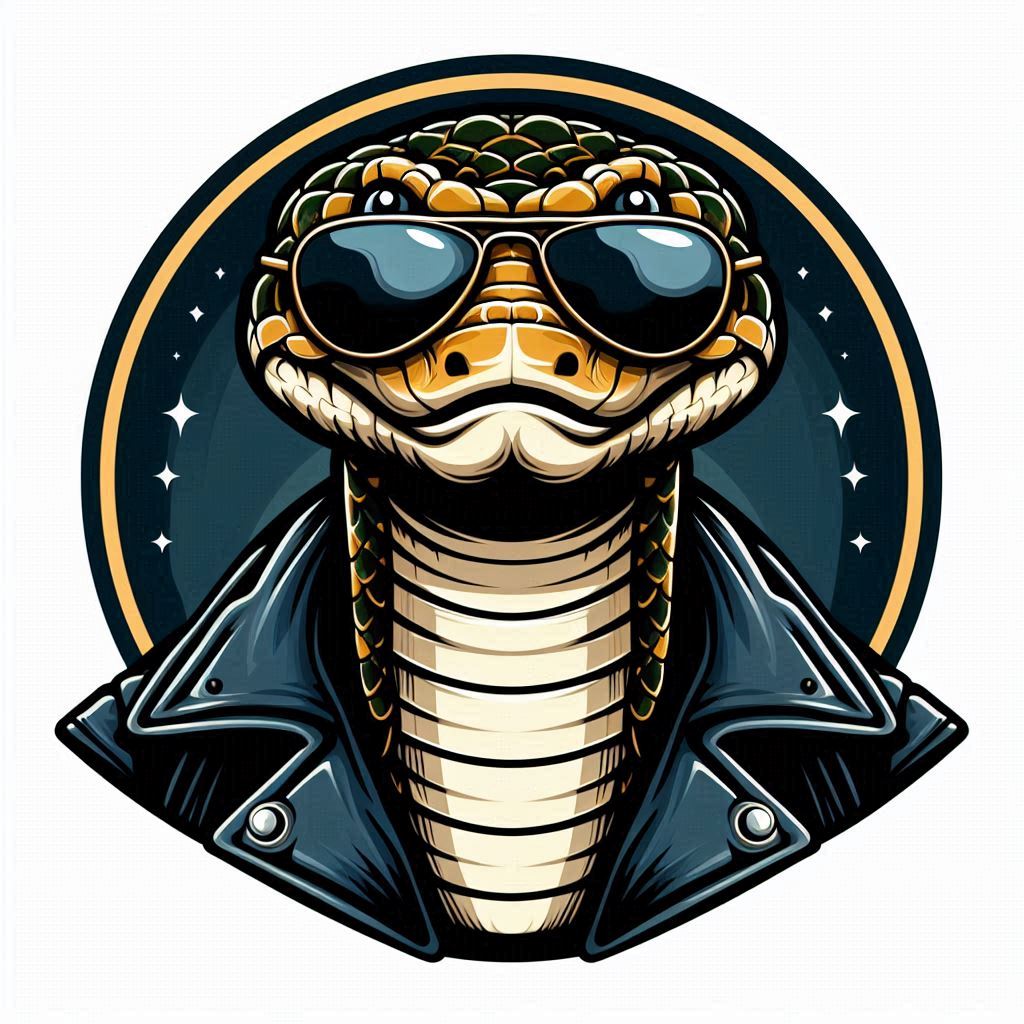
DRY Principle
August 10, 2024In the ever-evolving landscape of software development, choosing the right programming paradigm is crucial for building scalable, maintainable, and efficient code. Two of the most prominent paradigms are Object-Oriented Programming (OOP) and Functional Programming (FP). Each has its merits, but when it comes to adhering to one of the most important principles in software development—DRY (Don’t Repeat Yourself)—Object-Oriented Programming often shines brighter.
The DRY Principle: A Quick Recap
DRY, an acronym for "Don’t Repeat Yourself," is a principle aimed at reducing the repetition of code. The idea is simple: any piece of knowledge should be represented in a single place in your codebase. DRY promotes cleaner, more maintainable code by ensuring that changes or updates need to be made only once, in one location. This reduces errors, simplifies debugging, and makes your code more readable.
Object-Oriented Programming and DRY
Object-Oriented Programming (OOP) is centered around the concept of “objects,” which are instances of classes. Classes encapsulate data for the object and methods to operate on that data. OOP allows for the creation of complex systems that are easier to manage and extend.
Encapsulation: OOP promotes encapsulation, a core tenet where data and methods that operate on data are bundled together within classes. This structure inherently reduces repetition. For example, if you have a class `Car`, all attributes like `speed`, `color`, and `model` are encapsulated within the class. Any behavior related to a car, like `drive()` or `stop()`, is also included within the same class. This encapsulation ensures that any changes to car behavior or attributes only need to be made in one place.
Inheritance: One of the most powerful features of OOP is inheritance, which allows classes to inherit attributes and methods from a parent class. This promotes code reuse and significantly reduces redundancy. If you have a `Vehicle` class, a `Car` class can inherit from it, automatically acquiring all its properties and methods. This eliminates the need to rewrite code, staying true to the DRY principle.
Polymorphism: OOP allows different classes to define methods that are specified in a parent class, enabling the same method to operate differently on different objects. This flexibility reduces the need for repetitive code and switch-case or if-else statements often found in functional programming.
Functional Programming and DRY
Functional Programming (FP), on the other hand, is a paradigm that treats computation as the evaluation of mathematical functions. FP avoids changing state and mutable data, focusing instead on the application of functions.
Pure Functions: One of FP's core concepts is the pure function, which always produces the same output for the same input and does not cause side effects. Pure functions can enhance code clarity and predictability, contributing to a DRY codebase. However, FP tends to encourage smaller, more granular functions, which can sometimes lead to repetitive code if not carefully managed.
Higher-Order Functions: FP utilizes higher-order functions, which can accept other functions as arguments or return them as results. This promotes code reuse and can help achieve DRY. However, the trade-off is that FP's approach may result in more complex codebases where it becomes harder to track where and how different functions are applied.
Immutability: In FP, immutability is a core principle where data structures, once created, cannot be altered. This promotes safer and more predictable code but may lead to situations where similar transformations or operations are applied repeatedly to different data sets, potentially violating the DRY principle.
Why OOP Wins for DRY
While Functional Programming has its strengths, particularly in scenarios requiring pure functions and immutability, Object-Oriented Programming often provides a more straightforward path to adhering to the DRY principle.
OOP's encapsulation, inheritance, and polymorphism directly support the DRY principle by reducing the need for repetitive code. By organizing related code into classes and allowing objects to share behaviors through inheritance, OOP ensures that changes and updates are more manageable, reducing the risk of errors and making the code easier to maintain.
Conclusion
Both OOP and FP have their places in modern software development, and the choice between them often depends on the specific needs of the project. However, when it comes to maintaining a DRY codebase, Object-Oriented Programming typically offers more tools and structures to help developers avoid redundancy and write cleaner, more maintainable code.
By leveraging the strengths of OOP—encapsulation, inheritance, and polymorphism—developers can more easily adhere to the DRY principle, leading to better-designed software that is easier to understand, extend, and maintain over time.